There are plenty of different topics you’ll need to learn related to computer science and software development. Many CS courses don’t teach you a lot of real-world software development stuff, and some coding boot camps miss important CS concepts. This section aims to cover both.
The goal of sections 2-4 is to be a gentle introduction which is more palatable for beginners, whereas the later chapters build upon these general concepts and delve deeper into specific languages.
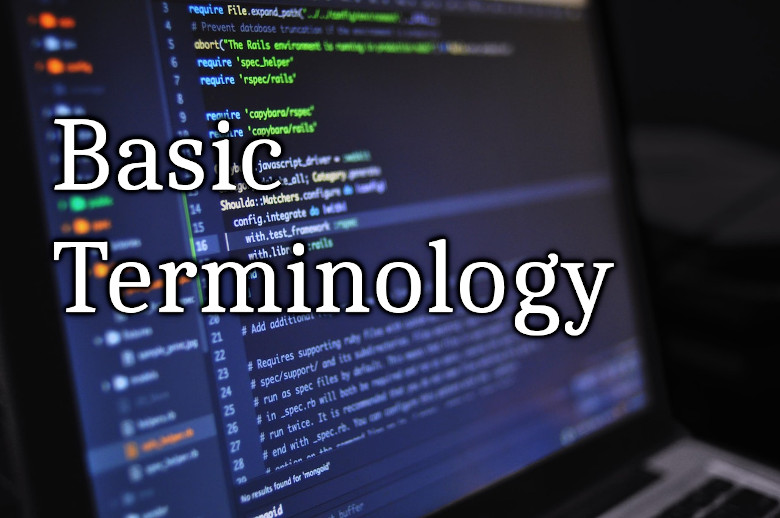
Programming – telling a computer what to do, using a combination of code and data. Programming can do all sorts of things, like loop, evaluate, save files, load files, perform if/then logic, define classes, create objects from said classes, create reusable pieces of code called functions, query databases and/or APIs, or use built-in features that someone else made, so you don’t have to reinvent the wheel.
Computer – there are many things that are computers, even if we don’t think of them as computers. Desktops, laptops, and tablets are all computers. Servers are computers. Routers are computers. Smartphones are computers. Cars have computers. Heating, ventilation, and air conditioning systems in buildings use computers and a special protocol called BACnet. Not all computers are personal computers. Computers used in industrial environments are often called embedded systems.
There are a few basic components of a computer, no matter what kind it is. If you’re going to be running or writing code on any kind of computing device, you might as well know the hardware.
RAM – fast, non-persistent storage. It stands for Random Access Memory. A computer will have less RAM than storage. When the device is powered off or crashes, it loses what was stored in RAM. Memory that is non-persistent is also called volatile memory.
ECC RAM – Error-Correcting Code RAM. Long story short, it’s more reliable than regular RAM, but it’s also more expensive. It wouldn’t make much sense to splurge on ECC RAM for your laptop or desktop, but servers can definitely benefit from having ECC RAM. ECC only impacts reliability, not performance.
Storage – hard drives, flash drives, cloud storage, and SSDs, or Solid State Drives, allow you to save data so that it persists even when the power is off. Retaining data this way is called non-volatile memory. This is slower than RAM. Hard drives are also called disks, drives, HDs, or HDDs. A boot drive is an HDD or SSD that contains your operating system. You can have multiple storage drives in a computer. SSDs are fast and more expensive, but HDDs are slow and cheap (per unit of storage). A common combination for desktop computers is to have a fast, somewhat low-capacity SSD for the OS and apps, and then a slower, higher-capacity hard drive for things that don’t require fast speeds, such as videos or word documents.
There is also a weird form of memory called NVRAM, which stands for Non-Volatile Random Access Memory. But regular RAM is volatile, so non-volatile RAM sounds confusing at first. NVRAM is basically RAM with a battery that can keep it on so that it keeps what’s stored in it even when a device is powered off. This makes NVRAM more like a hard drive rather than RAM. Routers commonly have NVRAM to store settings. If there is a problem with your router’s settings, you can press a button to clear the NVRAM, which will reset it back to default settings. Most of the time, you will only be dealing with regular RAM or storage such as HDDs or SSDs. Not NVRAM.
CPU – Central Processing Unit. It’s the “brain” of the computer, doing calculations and executing instructions. CPUs have different architectures, such as x86 or ARM. A CPU might have multiple cores, and even an integrated GPU.
GPU – Graphics Processing Unit. Your device will either have an integrated GPU, which is cheaper, or a dedicated graphics card, which is faster and more expensive. It’s what does graphics processing, such as for video rendering or 3D graphics. You don’t need a good GPU for coding.
Graphics cards have something called VRAM, which means Video Random Access Memory. VRAM is used for things like 3D graphics in video games.
IO – input and output ports on a computer, such as USB, headphones, ethernet, and more.
Networking – the ability for a computer to talk to other computers. It might be wired or wireless.
Power supply – converts alternating current (AC) from the wall outlet to direct current (DC) for the device to use.
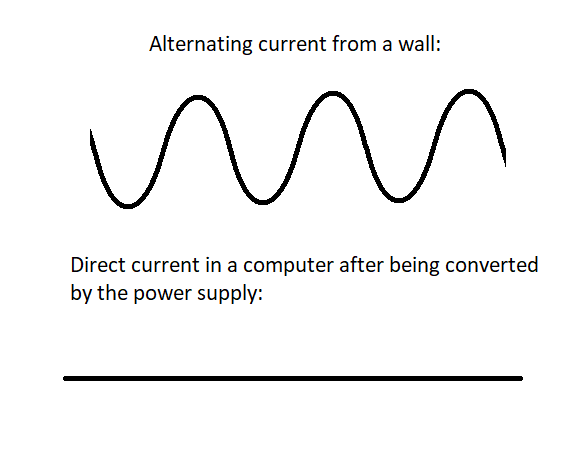
Motherboard, logic board, or main board – the board that all the parts connect into. It allows for the devices like RAM, CPU, etc. to communicate with one another using things called buses.
Heatsink and fans – some computer components get very hot, so they need to be cooled. Heatsinks dissipate heat from things like CPUs and GPUs. A heatsink with two fans is said to be in “push/pull” configuration because one fan is pushing cool air into it and the other is pulling hot air away from it. However, most computers will just have either just a push or pull setup.
If something has a heatsink and no fan, it’s called passive cooling. Cooling with fans or water cooling is called active cooling. Passive cooling is not as effective as active cooling, but it’s used more for mobile devices, whereas desktops and servers tend to have active cooling.
If a device gets too hot, it might either shut off entirely or just force itself to run slower so that it won’t generate as much heat.
Swap/page – also known as swap file, page address extension (PAE), or paging, it’s using a storage drive as a slower version of RAM. macOS and Linux call it swap, whereas Windows calls it paging.
If your computer has 8GB of RAM, but you’re trying to use software that needs 9GB of RAM in total, what happens? Well, you might think it just couldn’t run it. But actually, your operating system will use some of the free space on your hard drive or SSD instead. It’s not fast, but it’s better than running out of RAM entirely. Just keep in mind that performance will take a huge hit when you have to use swap/page.
File systems – the way to format a hard drive or solid state drive so that a computer can figure out where files are, or where free space is for putting new ones. A Windows file system might be something like NTFS. A flash drive can be formatted with FAT32 or EXFAT file systems. Linux distributions might use btrfs, ext4, or something like that. macOS uses HFS+ or APFS. A file server might use ZFS. You might occasionally see “file system” referred to as FS, such as /etc/fstab in Unix, which is short for file systems table.
Operating system (OS) – the software installed on the computer’s storage that lets you run apps. Some OSes include Windows, Android, iOS, Linux, and macOS. The operating system on Macs used to be called Mac OS X, but Apple recently rebranded it as macOS. You can tell that a book is outdated if it still says Mac OS X.
Computers used to run programs directly, with no OS. But you could only run one program at a time, making them very cumbersome and limited in usefulness. An OS allows you to multitask, running multiple programs at the same time. I don’t get why some people think a specific OS is perfect despite incontrovertible evidence that all OSes have security vulnerabilities. For more information about security vulnerabilities for anything you can think of, check out https://www.cvedetails.com/ or https://nvd.nist.gov/.
Kernel – the heart of an operating system. The kernel is always running and does all sorts of important things. Linux is a kernel, not an OS. But things called Linux distributions use the Linux kernel combined with other things, such as shells, shell utilities, DEs (Desktop Environments) or WMs (Window Managers), and more. A particularly nasty type of malware is a kernel-mode rootkit.
Output devices – monitors and speakers/headphones.
Input devices – keyboards, mice, touchscreens, and even microphones.
BYOD – Bring Your Own Device. Some companies, especially startups, will let you bring your own laptop instead of using their own. It sounds appealing in some ways, but it makes security more complicated.
Hello world program – the most basic type of program you can write. It’s the first program you’ll make, and then you’ll get into more advanced stuff as you progress through your studies. All it does is display the message “Hello, world!” and nothing more.
Abstraction – you can drive a car without knowing how a combustion engine works. All you need to know is how to turn the wheel, change the gear, and use the pedals for breaks and gas. For code, you don’t have to know every single detail of it in order to use it. When you write a “hello world” program, how does it put the text on the screen? Well, the answer is very complicated and probably not even worth learning, so most people accept that kind of abstraction.
IDE – Integrated Development Environment. It’s where you write, compile, run, and debug your code. Back in the day, people used more separate editors and compilers, and some people still do from time to time. But nowadays, people use IDEs because they’re essentially swiss army knives for programming. You write a paper in a word processing program. You make a drawing or edit a photo in image editing software such as Photoshop or Krita. You write software in an IDE.
Compiler – most of the time, the code we write is human-readable but not computer-readable. A compiler changes the code you write into code a computer can understand and run. Sometimes, compiling can take a long time on your computer, especially if you have a slow processor or you’re working on a large project. Sometimes, people will use a faster remote server to compile things. Sometimes, when you are working on a big project, you might only want to recompile a small portion of it that you changed instead of recompiling everything, which can take a lot longer, and might not even be necessary if you didn’t change a file. Linking, loading, and object code are related terms, but compiled languages are not the focus of this book. I think interpreted languages are better for the most part. But Java is also nice even though it’s not a compiled language. Java compiles to something called bytecode, which can be run on the Java Virtual Machine, regardless of the operating system it’s running on.
You’d assume that your compiler compiles your code regularly, translating source code into something your computer can run, but there are two notable examples of how compilers can’t always be trusted. One was the Ken Thompson compiler hack, where he made his compiler put a backdoor in all code compiled with it. A more recent example is the XcodeGhost compiler malware. You can trust the source code you write, but do you know what your compiler is doing behind the scenes? Many compilers are trustworthy, but it’s still an exciting topic of discussion.
I’m not trying to make you paranoid by listing these security things, but I am trying to make people more mindful about security and cautious when using software.
Sometimes I feel a little apprehensive about compiling/building a project because I think there’s a possibility that an issue can come up, such as a compile-time error because of a mistake I accidentally made. But if it finishes building successfully, I feel a wave of relief. If there is a compile-time error in your code, you will have to fix it before you can compile and run your code. Some errors are run-time rather than compile-time though.
Interpreter – an alternative to a compiler is an interpreter. You can run your code after writing it without having to compile it. That means there is less time between writing code and being able to see what it does, which can be very useful for debugging and testing (and just building incrementally). It does, however, sometimes mean that the performance of the running code is worse than that of a compiled program. Python is an interpreted language, whereas C++ is a compiled language.
GUI – graphical user interface. In Java, you can use something like Swing, JavaFX, or OpenJFX to add a GUI to your software. In Python, you can use Tkinter to make your program graphical. Other GUI frameworks include Qt and GTK, though they are more complicated. If you make a web app, you can use HTML, CSS, and JavaScript to make the GUI, typically using an additional library or framework like Bootstrap or Vue. Your first programs will be text-based, but eventually, you might want to make graphical programs.
The first graphical programs you make might look tawdry, but that’s okay. In many cases, software doesn’t need to be aesthetically-pleasing, so long as it’s functional and relatively intuitive to use.
Logic gates – gates that allow for logic to be carried out. They typically take two inputs and give an output. They use true or false, also called Boolean logic, named after George Boole. If you take a class that involves logic gates, you will probably have to write out truth tables, which show all the combinations of outputs based on the two inputs.
Some examples of logic gates include AND, NAND, OR, XOR, and NOT. AND and OR are simple ones. AND will only output true if both inputs are true, otherwise it will output false. OR will output true if either one of the inputs are true. XOR is exclusive OR, meaning it will only output true if only one of the inputs is true. NOT is a special kind of logic gate that only takes one input and outputs the opposite of it. So NOT true is false. NOT false is true.
Outside of computer science classes, you aren’t likely to use logic gates in the real world, at least if you’re getting into software development as opposed to some other field. However, you will be using the logic concepts, even if you’re not create circuit diagrams with logic gates.
In university, computer science majors will often have to take a class such as discrete structures, which will go over logic and truth tables. But even non-CS majors might have to take a logic-related class, such as a philosophy class, maybe called something like “reasoning and argumentation.”
Built-ins – pieces of code that you can use for free without
having to write it yourself are called built-ins. For example, you
can write System.out.println("hello
world");
without making the println()
function yourself in Java.
The difference between built-ins in a programming language and third party libraries you can download online is that there might be copyright stuff that might limit how you can use the third party code. Built-in features in a programming language are more lenient in how you’re allowed to use it, so it’s best to try using built-in stuff instead of immediately trying some third party solution.
print()
– a python function for displaying text in a terminal or console.
You can print strings or even variables.
Boilerplate – code that is entered in a lot. You will notice that some languages have a lot of boilerplate, such as Java. To make a simple hello world program, you need to do a lot.
Here is an example of boilerplate in Java (the main method in the Main class, which is the entry point of most Java programs):
public class Main{ public static void main(String[] args){ System.out.println("Hi"); } }
Comment – sometimes, in code, you want some plain English words that explain what your code does. They aren’t instructions or data. The computer ignores the comments. They are only there so you can read them later. When you’re writing code, you might think to yourself, “I’ll remember this later.” But you really won’t. You might as well write comments to explain what your code does.
//This is a single-line comment in Java
<!-- This is an HTML comment-->
#this is a shell script comment or Python comment
Sometimes, a comment isn’t a message for documentation. Every now and then, you might want to disable parts of your program, for whatever reason. Instead of deleting everything and losing it completely, you can just “comment it out,” meaning you turn a block of code into a multi-line comment. Many IDEs even support this feature within a right click menu or keyboard shortcut, to comment or uncomment highlighted text. I comment out code quite a lot.
Standard library/API – the standard library of a programming language, sometimes called an API (Application Programming Interface), includes many things you can add to your program without having to either write them yourself or use a third party library. When you choose to use an API, you are delegating certain things in your software to that API so that you don’t have to do it yourself. Sometimes, they are referred to as APIs, though API can have a different meaning, meaning something that you use to interact with a service in a limited way without seeing their proprietary intellectual property/secret sauce (such as the Twitter API). In Python, the Python Standard Library has a lot of good stuff worth looking into. It’s good to read the documentation to see what the language offers because sometimes you need something that you didn’t know exists. In Java, the default stuff you get is called the Java API from Oracle. Oracle didn’t make Java, but they bought it from Sun Microsystems. So sometimes, with Java, you might see the name “Sun” come up, but it generally refers to the old stuff. Oracle develops modern Java.
Keep in mind that local programming-related APIs are very different from remote web APIs. “API” means different things in different contexts.
Include/import – if there’s something in your programming language’s library, it won’t be put into your program until you use an include or import statement.
Let’s say you want to use Pi for a calculator app in Python. Then you’d do this:
from math import pi
Alternatively, if you want to import everything from math, do this:
import math
You can import things in the default library, or you can download third party stuff, but you should use the first party stuff whenever you can.
In C/C++, it’s like this:
#include <iostream>
Reserved words – in a programming language, there might be words you can’t use for things like names of variables/functions/classes. Some examples of reserved words could be import
, new
, delete
, if
, or while
. They are reserved because they serve a purpose in the language itself.