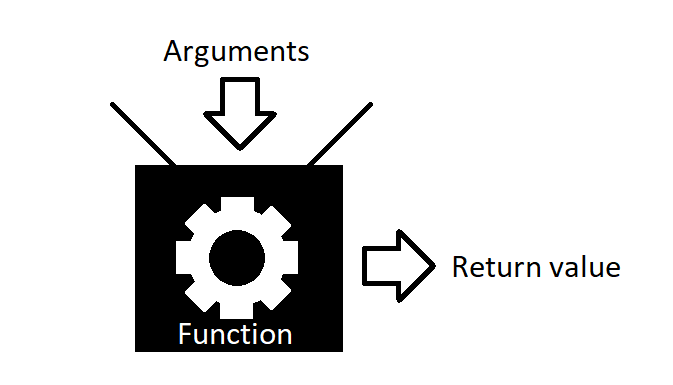
Function – a piece of reusable code. It can take input in the form of arguments and optionally return a value. These can be considered small, modularized sub-programs sometimes called subroutines. These sub-programs coalesce to form a bigger and more capable program. If you find yourself copying and pasting a lot of similar code, you might want to consider putting it into functions. Then all you have to do is call the function. You call, or invoke, a function using its name and any arguments for it.
Argument – a value passed to a function. Let’s look at the following Python example:
print(“hello”)
print() is the function, and “hello” is the string argument for it. A function is like a machine, and an argument is something you put into the machine. The function then performs actions, and can optionally return a value (the output of the machine).
Default arguments – if no argument is provided to a function, it can use a default value, called a default argument. The default argument is specified in the function header, not in function calls.
Return value – Let’s say you have a function called make_pie(). It returns an instance of the Pie class. In some cases, such as in Java, you have to explicitly define the type of return value of a function, during the function declaration. But other languages, such as Python, don’t need it. If something doesn’t have a return value, it uses a void return type, meaning it doesn’t return anything.
Another example:
int myNumber = add(2,5);
In the above example, you are taking the return value from a function and then assigning it to a variable called myNumber.
You don’t always need to do something with a return value, but they can be useful for many things. Some functions don’t have any return values at all, but a return type of void still has to be specified in the function definition.
Invocation or calling – a way of using a function. You write the name followed by the arguments in parentheses, separated by commas if there’s more than one. If there is a return value, it is given to the caller. The thing that calls a function is called the caller. An example of a function being invoked is as follows:
person_instance.set_first_name(“Joe”)
Nesting – when you put something inside something else, it’s called nesting. If you have a loop inside another loop, it’s a nested loop.
Modularity – the design principle of breaking up your code into smaller pieces that do things with a narrower scope. When you have a gargantuan and vague problem, you need to turn it into small and specific problems which are more manageable. Only then can you write your code.