C++ GUI options:
If you want to make a graphical program in C++, use the Qt library. You can get it at https://www.qt.io/
str.at():
#include <iostream>
using namespace std;
int main() {
string example = “whatever”;
for (int i = 0; i < 2; i++) {
for (int j = 1; j <= 2; j++) {
cout << example.at(j);
}
}
return 0;
}
Here is the output of the above program:
haha
The .at() function for a string in C++ will return a char at the given position within the string.
str.length():
Although there’s no length() method or property for arrays, there is a length() function for strings in C++.
#include <iostream>
using namespace std;
int main() {
string example = “whatever”;
cout << example.length() << endl;
return 0;
}
str.find():
#include <iostream>
using namespace std;
int main() {
string example = “the quick brown fox jumps over the lazy dog”;
cout << example.find(“over”) << endl;
//”over” is in the string
cout << example.find(“invalid”) << endl;
//if the return value of .find() is greater than the length of the string,
//then it means it’s not in the string
return 0;
}
C++ auto keyword (static type inference):
#include <iostream>
#include <typeinfo>
using namespace std;
int main() {
int x = 6;
auto y = x + 2;
cout << y << endl;
cout << typeid(x).name() << endl;
cout << typeid(y).name() << endl;
return 0;
}
C++ command line arguments:
#include <iostream>
using namespace std;
int main(int argc, char* argv[]) {
cout << “Hello, ” << argv[1] << endl;
return 0;
}
Here is an example of using the above main.cpp file with a command line argument:
$ ./chapter6_demos.exe Robert
Hello, Robert
C++ sleep:
Want to add a pause in your program to make it happen slower? Use Sleep() on Windows or usleep() on Unix-based OSes! There are other ways of doing this too, such as std::this_thread::sleep_for(), which requires #include <thread>. But let’s just keep it simple for now.
On Windows, Sleep() will sleep for a given number of milliseconds. There are 1,000 milliseconds in a second. Sleep(1000) on Windows will sleep for a whole second. Unix is a little weirder, because you can only use sleep() to sleep for entire seconds, such as sleep(1) for one second, or usleep() for microseconds. There are a million microseconds in a second, so to sleep for half a second using usleep, you’d have to do usleep(500000). The u in usleep is is supposed to look like μ (micro a.k.a. mu), which means one millionth. But you can’t use Unicode characters for identifiers, so they had to use u instead.
Here’s how to sleep on Windows:
#include <iostream>
#include “winbase.h”
using namespace std;
int main() {
cout << “Going to sleep…” << endl;
Sleep(2000);
cout << “Woke up from sleep!” << endl;
Sleep(1000);
cout << “Let’s slowly count multiples of 5” << endl;
Sleep(1000);
cout << “This demonstrates both sleep() and a different “;
Sleep(1000);
cout << “amount for incrementing an iterator in a for loop.” << endl;
Sleep(1000);
for (int i = 5; i<= 50; i += 5) {
Sleep(500);
cout << i << endl;
}
return 0;
}
Here’s how to sleep in Unix (Linux and macOS):
#include <iostream>
#include <unistd.h>
using namespace std;
int main() {
cout << “Going to sleep…” << endl;
usleep(2000000); //2 seconds
cout << “Woke up from sleep!” << endl;
usleep(1000000); //1 second
cout << “Let’s slowly count multiples of 5” << endl;
usleep(1000000);
cout << “This demonstrates both sleep() and a different “;
usleep(1000000);
cout << “amount for incrementing an iterator in a for loop.” << endl;
usleep(1000000);
for (int i = 5; i<= 50; i += 5) {
usleep(500000); //half a second
cout << i << endl;
}
return 0;
}
Hexadecimal numbers in C++:
You can use hexadecimal instead of decimal in C++. For example:
#include <iostream>
using namespace std;
int main() {
int hex_test[3] = {0x1, 0x10, 0x100};
for (int i = 0; i < 3; i+=1) {
cout << hex_test[i] << endl;
}
cout << 0xdeadbeef << endl;
return 0;
}
0x is the hexadecimal prefix, which means that the number after it is hexadecimal rather than decimal. But when you print it out, it shows the value in decimal.
Here is the output of the above program:
1
16
256
3735928559
Because hexadecimal is base 16, 0x1 is just 1 because it’s the ones place. Then 0x10 is 16, because it’s 1*16 and 0*1. 0x100 is 256 because it’s one place over from the 16s place, so it’s the 16*16s place. Hexadecimal numbers can sometimes be made to look like words, such as in 0xdeadbeef. Because hexadecimal uses 0-9 and A-F, there are a few words that can be made. 0xdeadbeef is a big number in decimal though.
Makefile – an old-school build automation configuration file. Makefiles are associated with make and C/C++.
Make – an older build automation tool. If you’re working on a C/C++ program, it might have many different files all in the same project. In order for the program to reflect the changes you’ve made in the code, you will need to recompile edited source code files and then do linking and loading. Make makes it easier to “make” the executable from multiple source code files. It’s a rudimentary form of automation.
In newer languages, you can ignore compiling entirely, or in the case of Java, you can just click the “build” button in your IDE and it does everything for you. But this wasn’t the case back in the day.
For more information about C++, check out http://www.cplusplus.com/
Congratulations on completing section 6!
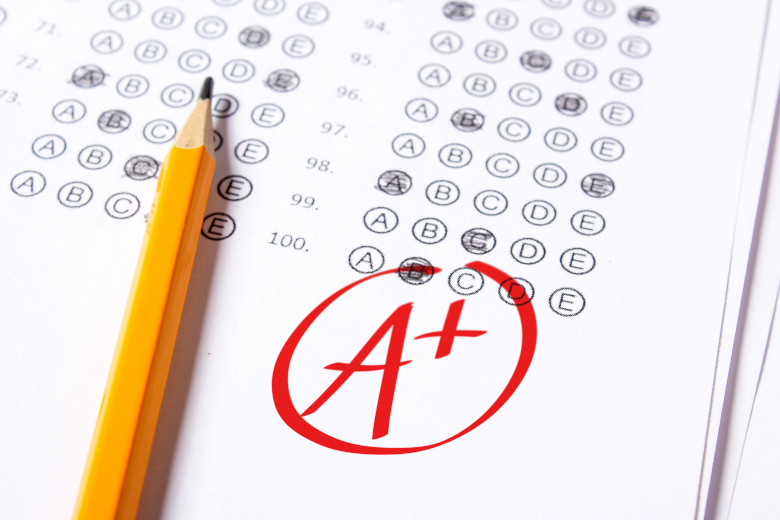
You’re now a C++ developer! You should feel proud of your educational accomplishment. C++ definitely isn’t the easiest programming language to learn.