- JavaScript – JS determines the interactivity of a website, at least on the frontend side of things (with a couple of exceptions like Node and MongoDB showing JS or JSON aren’t just for frontend uses). Very few people only use plain old JavaScript. Most people use some framework or another, such as Angular, React, jQuery, Vue, or seemingly countless others. It’s not a good idea to try and do input validation via end user JavaScript because someone can always disable JS in their browser. You need to validate things server-side instead.
- Some people criticize JavaScript and modern web development as a whole for being too slow or collecting too much personal information about users. And despite the names sounding similar, Java and JavaScript are not related.
- While languages like C++ or Java might require downloading and then executing a program on a computer, JavaScript is the language that web browsers use. So if you want something to be accessible to pretty much all devices, whether they’re computers, tablets, or phones, it should be a web app. JavaScript is the interactivity of a web page. Go to a website and the JavaScript can run automatically, sandboxed in the browser and able to run without having to be installed. It is often referred to as JS. JavaScript can either be embedded in a page directly, which is not ideal, or you can link to a .js file, using something like this:
<script src=”../js/script.js”></script>
- In more recent years, JS has been used for things aside from frontend interactivity, like server back-end business logic using the server software called Node. There is also a data exchange format called JSON, or JavaScript Object Notation, which is based on the Object Notation of JS. NoSQL/document-based databases like MongoDB look a lot more like JavaScript, unlike relational databases.
- Some people block JavaScript in their browser for privacy and security reasons. It’s true that some JavaScript can be malicious. There are things like JS-based cryptocurrency miners, which will make your CPU usage skyrocket to make money for someone else. There are also things called browser lockers, which are a type of scam that makes it hard or impossible to close your full-screen browser and demands that you pay money. Sometimes, browser vulnerabilities can be used in conjunction with JavaScript to deliver more nefarious payloads. In recent years, there have been JavaScript-based exploits for more obscure and complicated things like the Rowhammer bit-flipping attack, as well as security bugs in Intel processors, related to features like Intel’s speculative execution, which is found in a lot of modern processors.
- But that being said, most JavaScript is relatively benign, or at least won’t give you malware. A lot of JS is used for basic interactivity and layout, though it can also be used for less desirable non-malware things, like advertising or tracking. But if you disable JavaScript in your browser, most websites will look broken, and many won’t load properly.
- If you use my website template, you can easily make your own JavaScript within the script.js file, save it, and then refresh your browser tab/window in order to reflect the changes you made.
- If you want to check your JavaScript code and make sure it’s error-free, use this website:
- https://www.jslint.com/
- If you use JSLint, I suggest checking the following options first, under the “Assume…” section:
- in development
- a browser
- If not, you will get a lot of weird-looking warnings and errors that don’t make sense for the context of web development.
For the following brief JS examples, create an HTML page called test.html, with the following code:
<!DOCTYPE html>
<head>
<meta charset=”utf-8″>
<title>JS Test</title>
<script src=”test.js”></script>
</head>
<body>
</body>
</html>
- And create a blank JS file called test.js in the same folder as the test.html file. Use the following JS examples in the test.js file. Open/refresh the test.html file in a browser, such as Chrome or Firefox.
- To view the output for the following examples, in Chrome, in the test.html tab, right click on the page and hit “inspect,” then hit “console.” This is where the output of the following JS examples will be. You will need to refresh the page every time you make a change to the .js file in order to see the output in the console.
- To view console output in Firefox, open test.html in Firefox, then right click and hit “inspect element.” Then click the console tab.
- JS variables:
- Write the following code in the test.js file, save it, and then view it in a browser tab (refresh it if you already have it open, and then view the console again using the above instructions):
var message = “hello”;
console.log(message);
var number = 5;
console.log(number * 2);
- JS operators and operands:
var message1 = “this”;
var message2 = “that”;
console.log(message1 == message2);
var num1 = 5;
var num2 = 7;
console.log(num1 + num2);
num2 += 3;
num2 *= 5;
console.log(num2 % 2);
num1++;
num2–;
num1 -= 20.0;
console.log(num1/num2);
console.log(num1);
console.log(num2);
console.log(num1 > num2);
console.log(num1 <= num2);
console.log(5 != num2);
console.log(5 % 2 == 0 || 60 % 2 == 0);
num1 = “not a number anymore”;
//js is dynamically-typed
console.log(num1);
- JS functions:
function add(num1, num2){
return num1 + num2;
}
var sum = add(100, 200);
console.log(sum);
- JS control flow:
var num1 = 75;
var num2 = 23;
if (num1 > num2) {
console.log(“num1 is the greatest”);
} else if (num1 == num2) {
console.log(“num1 and num2 are the same”);
} else {
console.log(“num2 is greater than num1”);
}
- JS exception handling:
var x = “example”;
try {
//can’t add 1 to a string
//it will result in NaN
//which means Not a Number
if (isNaN(x + 1)) {
throw “NaN error”;
}
} catch (e) {
console.log(e);
}
- JS classes and objects:
class Person {
constructor(firstname, lastname) {
this.firstname = firstname;
this.lastname = lastname;
}
introduce() {
return “Hi, my name is ” + this.firstname + ” ” + this.lastname;
}
}
let paul = new Person(“Paul”, “Smith”);
console.log(paul.introduce());
- JS input and output:
- Output to the screen:
console.log(“hi”);
- User input:
var color = window.prompt(“What is your favorite color?”);
console.log(“Your favorite color is ” + color);
- JavaScript changes something called the DOM, or Document Object Model, which is an API that is used for dealing with HTML. You can add things, change things, delete things, search for things, and more. You can interact with every part of a web page using JavaScript and the DOM.
- Here’s an example of DOM manipulation in JavaScript:
document.writeln(‘<h1>This text is big!</h1>’);
- ln is short for line. So it’s writing a line of text on the web page.
- If you view the HTML page that is associated with that JS file, you will notice that it doesn’t just show the text literally. You can add HTML with it. It will show up as a new <h1> tag, so the text will be big.
- The web page is the document. Sometimes, though, it’s not enough to write something to the document generally. You might want to specify a particular part of the web page to put something in.
- My website example has a projects.html page that has a feature that will show you random quotes from a list of quotes, so long as you press a button.
- Here’s the JavaScript code for it:
console.log(“You’re viewing the console! Good job!”);
var quotes = [
“Here is a cool quote”,
“Here is another cool quote”,
“Here is yet another cool quote”,
“This is the 4th quote”,
“These are randomly picked”,
“Quote 5”,
“You can become a software developer”,
“Customize this site and make it your own!”,
“Software development is for everyone!”
];
var authors = [
“Author 1”,
“Author 2”,
“Author 3”,
“The 4th Author”,
“Some Random Author”,
“Person 5”,
“Wise person”,
“Alan”,
“Someone”
];
var count = 1;
var numberOfClicks = 0;
function newQuote() {
var randomNumber = Math.floor(Math.random() * quotes.length);
document.getElementById(“quoteText”).innerHTML = “\””;
document.getElementById(“quoteText”).innerHTML += quotes[randomNumber];
document.getElementById(“quoteText”).innerHTML += “\””;
document.getElementById(“quoteAuthor”).innerHTML = authors[randomNumber];
count = count + 1;
document.getElementById(“quoteCount”).innerHTML = “Number of quotes”;
document.getElementById(“quoteCount”).innerHTML += “shown: “;
document.getElementById(“quoteCount”).innerHTML += count;
console.log(“newQuote() function was called”);
}
function countClick() {
numberOfClicks = numberOfClicks + 1;
console.log(“You have clicked the page ” + numberOfClicks + ” times.”);
}
function exampleAlert() {
alert(“Here is a JavaScript alert!”);
}
- The var keyword is for any type of variable. JavaScript is similar to Python in the sense that it’s dynamically-typed, whereas Java is strongly-typed. Java has a var keyword, but it’s only used for static type inference, as opposed to dynamic typing. Recall that dynamic typing means you can have a variable assigned to one data type and then reassign it to something else. Static typing means a variable can only be a certain kind of thing, and if you want to change it, you need to use a typecasting function, which returns a different data type. Weakly typed means you’re not spelling out what the data type is. Strongly typed means you always have to specify what something is. JavaScript is a dynamic, weakly-typed language. Java is a strong, statically-typed language. JavaScript is usually run in a web browser, whereas Java is used for native programs and server-side stuff. Despite sounding similar, you can see that they are very different.
- Strong typing means you have to tell the computer what the data type is, such as int or string. Weakly typed means the computer will figure out what kind of data type it is even if you don’t specify it.
- For var quotes and var authors, these are examples of arrays in JavaScript. They have multiple elements, each accessible with their corresponding index, starting with 0. Always start counting with 0 in computer science. I could have also used a list instead, but I’m just keeping it simple for now.
- var count = 1 is used to keep track of the number of quotes that are displayed on the projects page. This will reset every time you refresh the page. If you want persistence, you will have to use a database or cookies. But those are above and beyond the scope of this book.
- There are two buttons on the projects page:
<button onclick=”newQuote()”>New Quote</button>
<button onclick=”exampleAlert()”>Alert Example</button>
- The JS file is included in the page with this code:
<script src=”js/script.js”></script>
- The onclick attributes in the button elements correspond to function calls. The var count is used to keep track of quotes, and var numberOfClicks = 0; is used to keep track of the number of times the user clicks on the page. Keeping track of clicks on a page is pretty much pointless, but I made it this way to demonstrate some simple JavaScript concepts.
- Moving on, we have the function called newQuote. And I am using camel case here, but you might prefer to use a different naming convention. Camel case means the first word in the name is lowercase, and then all following words have the first letter capitalized.
function newQuote() {
var randomNumber = Math.floor(Math.random() * quotes.length);
document.getElementById(“quoteText”).innerHTML = “\””;
document.getElementById(“quoteText”).innerHTML += quotes[randomNumber];
document.getElementById(“quoteText”).innerHTML += “\””;
document.getElementById(“quoteAuthor”).innerHTML = authors[randomNumber];
count = count + 1;
document.getElementById(“quoteCount”).innerHTML = “Number of quotes”;
document.getElementById(“quoteCount”).innerHTML += “shown: “;
document.getElementById(“quoteCount”).innerHTML += count;
console.log(“newQuote() function was called”);
}
- This function takes no arguments, and instead, it just puts a new random quote on the page. It generates a random number and uses that as the index for which quote to put into the web page. notice one important DOM/JS concept here: document.getElementById(). With this method, I can get an element, using its ID (which I came up with), and then use the .innerHTML property in order to change it. I could have done the new stuff on a single line, but JSLint complained about my line being over 80 characters long, so I split it into multiple lines, which isn’t really necessary.
- After that, I also increased the count variable, which keeps track of how many quotes have been shown.
- This function by itself does nothing. Functions need to be called. A function call is also called an invocation. There is nothing in this JS file that invokes it. Instead, when the HTML page has a <script> tag to specify the src of the .js file, we are then able to use function calls for functions that have been written in that file. So that’s why the <button onclick=”newQuote()”>New Quote</button> button works.
- If you’re having trouble with your JavaScript, there is one thing you can do that might fix it (depending on the situation). If you move the <script src=”script.js”></script> file towards the end of the HTML document, such as in the footer, then everything else will have loaded first. HTML pages load from start to finish. Also, if you have multiple JS files, the order in which they are included can affect how things run in the page. This is especially important if you are using libraries and things that have dependencies. For example, Bootstrap requires jQuery, so you need to put the jQuery <script> tag before the Bootstrap JS and CSS ones.
- Something else you can try is the defer or async attributes for the <script> tag. For example:
<script async src=”../js/script.js”>
- defer means it will only run the script once the entire page has loaded. async means the script in question can be run asynchronously. It will run whenever it can, even if the rest of the page hasn’t loaded yet.
- The countClick() function increments the numberOfClicks variable and logs a message to the console.
function countClick() {
numberOfClicks = numberOfClicks + 1;
console.log(“You have clicked the page ” + numberOfClicks + ” times.”);
}
- In my example website, this will happen whenever you click somewhere on the web page. That only happens when you use the following code in the HTML page that includes this JS file with the countClick function:
<html lang=”en” onclick=”countClick()”>
- Everything will be within the <html> tag, so onclick in that tag means any time the user clicks on the page, even if they don’t click on a button element. Many other events can trigger JavaScript, such as onmousemove, click, dblclick, drag, cut, copy, keypress, keydown, and so on. For more DOM events, look up the DOM event documentation on w3schools.com:
- https://www.w3schools.com/jsref/dom_obj_event.asp
- And also look at the DOM document object’s documentation here:
- https://www.w3schools.com/jsref/dom_obj_document.asp
JS has a lot of standard fare programming concepts, but it also features some browser-specific things. It’s got your usual functions, variables, if/then, for loops, comments, math, data types, while loops, try/catch, regular expressions, comparison, and so on. Nowadays, JavaScript supports classes, and with it, it supports class-based inheritance. If you read the previous chapters about programming concepts, you should be familiar with classes, objects, and inheritance. But older versions of JS used something called prototypical inheritance instead of class-based inheritance. However, I suggest sticking with newer versions and using class-based inheritance because I think it’s an easier concept to wrap your brain around.
- One feature I didn’t include in the website example is getting user input and using it to manipulate the DOM tree.
- Here is an HTML file that will correspond with the subsequent JavaScript file for a user input demonstration:
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”utf-8″>
<title>Test web page for JavaScript</title>
<script src=”program.js” defer></script>
</head>
<body>
<header>
<h1>User input example</h1>
</header>
<main>
<p>Hello there! This is a test web page</p>
<p id=”foodChoice”></p>
</main>
</body>
</html>
- And here is the user input JavaScript, called program.js:
userInput = prompt(“Enter your favorite type of food: “);
newValue = “Your favorite kind of food is ” + userInput;
document.getElementById(“foodChoice”).innerHTML = newValue;
- prompt() gets user input by creating a pop-up alert()-style box. You then take the return output from that function and then assign it to a variable. It takes the user’s choice and then changes the innerHTML value of a <p> tag with an id of foodChoice. Another way to get user input is with an <input> or <textarea> tag, and a button with the onclick attribute corresponding to a JS function that gets the text, using document.getElementById(“TextIdGoesHere”).value.
- Another quirk related to JavaScript is the concept of hoisting. In other languages, you will need to declare something before you can use it, like a variable or function. But in JavaScript, you can use a variable before you declare it – but it only works for out-of-order declarations – not initializations. It still needs to be initialized.
So here’s something you can’t do in another language:
x = 5;
alert(x);
var x;
- But the above example is perfectly valid in JavaScript, using hoisting.
- But the following would not work, even with hoisting:
alert(x);
var x = 5;
- There’s a lot more to JavaScript. This section is just intended to help you get acquainted with it, not to make you an expert on each and every facet of it. JavaScript: The Good Parts is an excellent book to learn JavaScript. But no amount of reading will make up for a lack of experience. Just start coding. It doesn’t have to be a huge app. Make a program that takes a number and adds one to it and then logs it to the browser console. Just start somewhere. Anything is better than not getting any real experience at all.
Congratulations on finishing section 10!
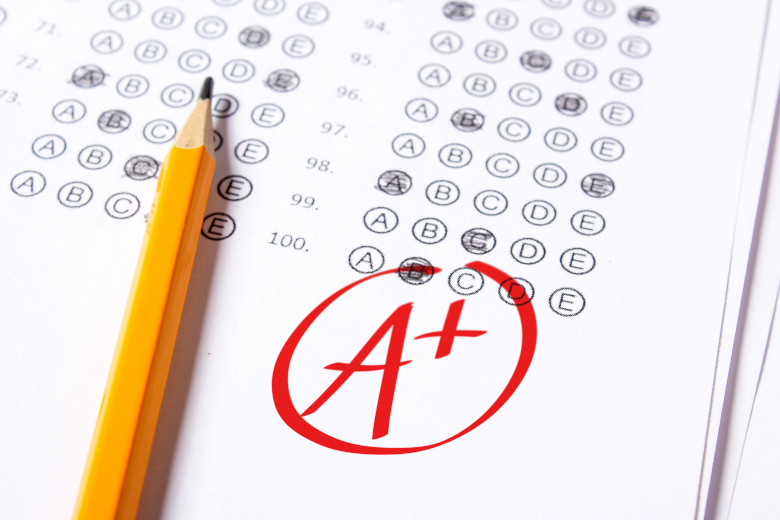
You now know enough to become a front-end web developer!