Programming Languages
You won’t be using every single one of these languages. It’s just good to be aware that they exist.
Machine code – the actual language of computers. It is not portable at all and unreadable by people — nobody codes in machine language. Machine code on one particular type of processor will be different from machine code on a different type of processor. By contrast, you can run C++, Python, or Java code regardless of what kind of processor it’s running on.
Assembly – a more low-level programming language. Lower than C or C++, but higher than machine code. Nobody codes in assembly these days, but you might learn it in a class if you study computer science in university. It’s not useful to know in the real world unless you get into reverse engineering or malware analysis.
Low-level programming does not mean low skill. Higher level languages like Python are easier to use. Low level refers to the level of abstraction. Assembly is closer to the hardware and involves manipulating registers and memory. Assembly can be difficult to use because you have to do many things manually, and it seems more arcane – stuff that sounds less human-like and more machine-like. Modern language concepts are easier to wrap your head around, but assembly requires better knowledge of hardware.
The XY problem – it’s relatively easy to make a website using PHP, but almost impossibly difficult to do the same thing in assembly. As such, rather than trying to come up with a very complicated solution to an unnecessary problem, such as writing a web server project in assembly, it might be better to just realize that the problem doesn’t need to exist in the first place (use PHP instead).
This phenomenon is referred to as the XY problem. “I want to do X, and I think I can do it with solution Y.” So you are trying to focus on how to do Y, rather than thinking about how there might be a better solution that isn’t Y. So if your problem X is that you want to make a website, Y would be trying to write it in assembly (which is a terrible idea). Looking for information on solving issues related to Y is the wrong approach. Maybe the real solution is Z (writing it in PHP instead).
C – the precursor to C++. C is a procedural language. A kind of archaic language by today’s standards, though much of the software we use today is still written in C. Linux is written in C, for example.
C++ – a language based on C, but with added features, most notably classes. It’s like C, but object-oriented. I am personally not a fan of C or C++. You have to deal with memory management, and there are some features like pointers which commonly confuse people. C++ also doesn’t hold your hand and will allow you to make big mistakes. For example, in Java, things need to be initialized. But in C++, it doesn’t care, and you can end up making mistakes that lead to security or stability problems. I believe that the onus should be on the language rather than the developer as far as this stuff goes. It is my personal belief that C++ makes it too easy to write insecure code. Newer languages, such as Java, Python, Ruby, or JavaScript, alleviate some of the issues of older languages like C and C++.
Perl
– an older language, sometimes used for web development
backend/business logic. It’s also used for short one-liner scripts
similar to shell scripts for doing simple tasks well. It was trying
to be what Python became, but isn’t as popular as it once was. If you
ever go to a web page and see .pl
in a URL, that means a Perl script is running – at least if it’s
something like example.com/post.pl
rather than something like example.pl
,
in which case the .pl
is a
TLD that means it’s a Polish website. One common criticism of Perl
is that it’s a very terse language that is hard to read.
Java – a language that was created to address some of the shortcomings of C++. It is still widely used, especially for backend business logic in large-scale enterprises. However, it has fallen out of style in certain ways. For example, there used to be browser-based Java applets, but they have been killed off because of all the security issues and how they were used for giving people browser-based malware (called a “Java drive-by download” or simply “Java drive-by”). Java is also being replaced with Kotlin for Android app development, but I think Java will still be around for a very long time. It is often used in CS academia as a first or second programming language. I’ve personally written a lot of software in Java, but I recognize the importance of other languages too.
C# – Microsoft’s language that is very similar to Java. Topics related to C# include ASP and .NET. If you’re all-in with Microsoft, C# might make sense to learn. Otherwise, Java is a better choice. But if you know Java, it’s not hard to switch to C#.
Python – one of my favorite programming languages. It’s excellent for learning programming. I recommend Python, Java, and JavaScript as the three languages people should start with. Well, and I suppose CSS and HTML also go along with JS. But you can use Python to write command line computer programs, graphical computer programs, and using Django or Flask, you can write full-stack web apps using Python too. It lets you concentrate on logic and your app rather than hardware-related stuff.
When you use Python, make sure you use Python 3. The current version might be 3.8 or 3.9 or something like that. There are still lots of things that use Python 2.7, but Python 2 is outdated and should be avoided. You will sometimes come across Python programs, libraries, or even tutorials that are for older versions of Python. You should avoid them.
There is some confusion with these two separate versions, and when you’re starting, you might accidentally use the wrong version of Python. Depending on your system and how you have Python installed, you might have to use either python or python3 at the command line.
Use the following commands to see your versions:
python --version
python3 --version
Also, only use Python 3. python sometimes corresponds to Python 2, but depending on your path variable, it might be set up for Python 3.
Ruby – an object-oriented programming language known for the Ruby on Rails web framework. It’s not as popular as it used to be, but some full-stack web developers still use it. Java and C# are often compared because of how similar they are. Ruby is often compared to Python, as they are both interpreted languages that attempt to make programming simpler compared to previous generations of programming languages (such as Java or C++). There used to be a Ruby vs. Python debate, but nowadays it’s clear that Python won that competition.
R – a programming language used for statistics and data analysis. I’ve never used it before, as it is a niche language rather than general purpose. By contrast, Java and Python are general purpose languages that can be used to make all different kinds of software. If you’re not into statistics, don’t bother with R.
Haskell – a functional programming language. Some functional programming concepts include lambdas, monads, and currying. This website doesn’t cover functional programming, except for the one example of lambda expressions being incorporated into primarily object-oriented languages.
Erlang – a functional programming language with enticing features such as self-healing: the ability for a program to modify itself while it’s still running.
Go – a language mostly used by Google and not many others.
Rust – a language kind of like C++ but with an emphasis on memory safety. C++ is a very unsafe language that makes it easy to write insecure code. Rust tries to fix these issues. A lot of Mozilla Firefox is written in Rust.
Objective-C – the programming language that used to be the primary way of developing iOS apps, but has mostly been replaced with Swift.
Swift – a programming language mainly used for writing iOS apps. Optionals, nil, and unpacking are concepts that pertain to the language. Swift is often used in Xcode, along with iOS Simulator and Interface Builder. One fascinating feature in Swift is that you can pass a function as an argument to another function, or even have a return value of a function. Swift was created in 2014, so it’s quite new. Compare that to Java, which was made 1995, or C, which was made in 1972.
An easy way to start messing around in Swift in Xcode is to use a Swift Playground.
Kotlin – just like how Objective-C is being replaced by Swift for iOS development, Android development used to be predominantly Java-based, but now Google is trying to replace it with Kotlin. Does that mean Java is dead? No. Kotlin is still a JVM language. JVM stands for the Java Virtual Machine. Simply put, Kotlin can’t exist without Java.
Although the specific features of Kotlin and Swift are different, I would still group them together because they are relatively new languages and they are both primarily used for developing mobile apps.
HTML, CSS, JavaScript, and SQL – with these programming and markup languages, and additional backend server software/languages such as PHP and the Apache web server, these are used for web development. More on that in the web development sections later on (if you’re reading this site in the suggested order of sections).
Pseudo-languages
There are some pseudo-languages I have learned, like regular expressions and SQL. They are very different from general-purpose languages like Python or Java, but still languages nonetheless. You understand what general purpose means once you get more into specialized things that can only do certain things. There are some pseudo-languages called sed and awk that are used for text manipulation, such as searching text, or substituting one thing for another within text.
Markup language – unlike a programming language, a markup language is just for displaying things. Markdown is a markup language. LaTeX is a markup language. HTML is a markup language. They can’t do all the interactive stuff that a fully-fledged programming language can do.
LaTeX – a markup language for document typesetting. It is commonly used in academia for research papers, dissertations, math formulas/notes, and even resumes. It is pronounced “law-tech.” Some people criticize it for not being very user-friendly compared to things like Microsoft Word. I’ve used it here and there, and while it’s not as easy to use as more modern options, you can still get the hang of it if you read the documentation about it. You can use MacTeX for macOS or TeX Live for Windows and Linux.
If you’ve ever read a research paper, such as from JSTOR, and you noticed that the document has a certain font and general aesthetic quality to it, it might just be made with LaTeX instead of Micrsoft Word.
Here is an example of a calculus limit law written in LaTeX:
\begin{equation*} \lim_{x \rightarrow a} \sqrt[n]{f(x)} = \sqrt[n]{ \lim_{x \rightarrow a} f(x)} \end{equation*}
Here’s what it looks like:
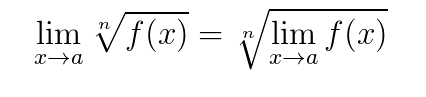
This
is how I took my math notes in college. I write them with pen and
paper and then later convert them to LaTeX markup. Anki is digital
flashcard software that has support for LaTeX, using the
[latex][/latex]
tag.
If you’re in academia, especially for advanced degrees as opposed to undergrad or community college, then use LaTeX. If you’re not, skip it.