- UML – Unified Modeling Language. Used for coming up with specifications for how a program and its classes should be like. You can list properties and actions as well as access modifiers, among other things. It’s an intermediary step between your first idea for an app and actually going and writing it. Just like how you don’t write a book start to finish before making some barebones structure first, you do similar planning before jumping right into coding.
- UML diagrams involve making class mock-ups and showing the relationship between them, such as for inheritance. The relationships between UML class diagrams are drawn with lines and directional arrows to indicate which is the superclass and which is the subclass.
- The format for a UML class diagram is as follows:
———————————–
NameOfClass
————————————
+public_attribute:type
-private_attribute:type
#protected_attribute:type
————————————
+public_function(arg1: argtype, arg2: argtype):return_type
#protected_function(arg1: argtype, arg2: argtype):return type
-private_function(arg1: argtype, arg2: argtype):return_type
- The top section is for the class name. The middle section is for attributes, which are also called instance variables. These are the things the class has. The bottom section is for class methods, which are things the class does. The attributes need to have their type specified after the colon following the variable name.
- +, #, and – are for access modifiers. + means public, # means protected, and – means private.
- Class methods need to have their arguments and argument types defined in parentheses. Then, they need to have a colon followed by their return type. Even if a method doesn’t return anything, it still has a return type (void). The only methods that don’t have any return type at all are constructors and destructors.
- Here is a UML diagram for a CuteCat class:
———————————–
CuteCat
———————————–
-numberOfLives:int
-isHungry:bool
-catName:String
———————————–
+CuteCat()
+CuteCat(lives:int, hunger:bool, name:String)
+meow():void
+setLives(numLives:int):void
+getLives():int
+setHunger(hunger:bool):void
+getHunger():bool
+setName(newName:String):void
+getName():String
The reason for using UML is because it’s easy to forget about ideas when they’re in your head, and it’s faster to write a UML diagram for a class than it is to write an entire class. Just like how you need an outline for an essay or a book, creating UML diagrams for your program can help you plan it out and work more effectively rather than just jumping right in with no planning ahead of time.
One thing you will notice about the CuteCat class is how it has getters and setters for every attribute. This is something you’ll see quite often in object-oriented programming, especially in computer science academia. In the real world, some people just make attributes public and then access class members directly. But they can be good some of the time. It’s definitely possible to overdo it though.
Congratulations on completing section 8!
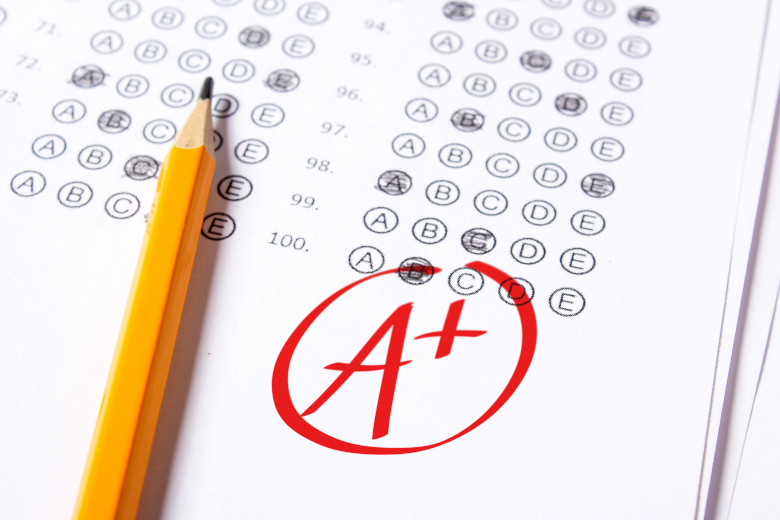
Version control is an essential skill for any software developer. You’re on your way to becoming a pro!